Functional techniques
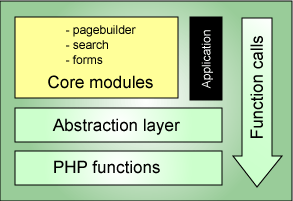
Using references
Simply put, references are just like pointers in C. The only difference
is that you don't need to de-reference them in PHP like you do with the
*
operator in C. You can think of them as an alias to a variable, array,
or object. Whatever operations you carry on, the alias affects the real
variable.
Listing 1 offers a look at an example.
Listing 1. Variable references
|
When you pass arguments to functions, the function receives a copy of them. Any changes you make to the arguments get lost as soon as the function returns. This is a problem if you want to alter the arguments directly. Listing 2 shows an example that demonstrates the problem.
|
We want $myNum
to be altered directly; this can be easily done by passing the reference of $myNum
to the half()
function. But remember, this is not a good practice. Developers who use
your code will have to keep track of the references used. This can be
one way bugs creep in unintentionally. It also affects how easy your
functions are to use .
A better practice is to use references directly in the function declaration -- in our case, half(&$num)
instead of half($num)
. This way you can intentionally forget to pass arguments to functions by remembering the reference.
PHP takes care things behind the scenes. Newer PHP versions (from 4.0 forward) deprecate call-time pass-by-reference and issue a warning regardless. (Some advice here: If you are using code made for older PHP versions, it's better to update it rather than changing PHP's behavior by altering the php.ini file.
Retaining variables between function calls
You often need to maintain the value of variables between function calls. Global variables can be used, but variables are vulnerable and may be corrupted by other functions. We want our variable to be local to the function and still retain its value.
Employing the static
keyword is an elegant solution. I often use this when I want to count
how many user-defined functions are executed on sites in which debugers
are not available. I simply alter all functions (using an automated
script, of course) and add a call to function that does the job of
counting on the first line of the function body. Listing 3 describes
the function.
|
Collecting the return value in a variable by calling funcCount()
just before the script finishes does the trick. Surprisingly, $count
is not reset to zero; the line that initializes the static variable is only executed once.
If you must access a global variable inside a function, you need to use the global
keyword before using the variable.
One more thing about starting from PHP 4 -- it's OK to use a function first and define it afterwards, provided you don't attempt to declare it twice.
Doing dynamic calls
In many situations you'll find that you really don't know which function has to be called next. Such situations arise when you are doing event-driven programming or when you want to call a particular function when an event external to your system has been triggered. Scripts that communicate over a network illustrate this situation.
The method is similar to using variable names. You simply use the external event to set a variable and use it as a function (provided you have declared corresponding functions). Confused? Listing 4 make things clear.
|
When you feel lazy, you can also use this method for writing several switch-case statements to evaluate which function to use. Simply set the variable and use it as a function. However, though we are deliberately setting variables here, remember that it can be done dynamically and that is what gives this technique its power.
View Develop Rock Solid Code In PHP: Part 3 Discussion
Page: 1 2 3 4 5 Next Page: Summary and Resources