Methods
Put simply, methods are functions declared within a class. They
are usually -- but not always -- called via an object instance using
the object operator. Listing 5 adds a method to the Dictionary
class and invokes it.
Listing 5. Adding a method to the Dictionary class
|
It provides output of:
|
As you can see, the summarize()
method is declared just as any function would be declared, except that is done within a class. The summarize()
method is invoked via a Dictionary
instance using the object operator. The summarize()
function accesses properties to provide a short overview of the state of the object.
Notice the use of a feature new to this article. The $this
pseudo-variable provides a mechanism for objects to refer to their own
properties and methods. Outside of an object, there is a handle you can
use to access its elements ($en
, in this case). Inside an object, there is no such handle, so you must fall back on $this
. If you find $this
confusing, try replacing it in your mind with the current instance when you encounter it in code.
Classes are often represented in diagrams using the Universal
Modeling Language (UML). The details of the UML are beyond the scope of
this article, but such diagrams are nonetheless an excellent way of
visualizing class relationships. Figure 1 shows the Dictionary
class as it stands. The class name lives in the top layer, properties in the middle, and methods at the bottom.
Figure 1. The Dictionary class shown using the UML
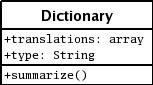
The constructor
The PHP engine recognizes a number of "magic" methods. If they are
defined, it invokes these methods automatically when the correct
circumstances arise. The most commonly implemented of these methods is
the constructor method. The PHP engine calls a constructor when the
object is instantiated. It is the place to put any essential setup code
for your object. In PHP V4, you create a constructor by declaring a
method with the same name as that of the class. In V5, you should
declare a method called __construct()
. Listing 6 shows a constructor that requires a DictionaryIO
object.
Listing 6. A construtor that requires a DictionaryIO object
|
To instantiate a Dictionary
object, you need to pass a type string and a DictionaryIO
object to its constructor. The constructor uses these parameters to set
its own properties. Here is how you might now instantiate a Dictionary
object:
|
The Dictionary
class is now much safer than before. You know that any Dictionary
object will have been initialized with the required arguments.
Of course, there's no way yet to stop someone coming along later and changing the $type
property or setting $dictio
to null. Luckily, PHP V5 can help you there, too.
View Getting started with objects with PHP V5 Discussion
Page: 1 2 3 4 5 6 Next Page: Keywords: Can we have a little privacy in here?